views
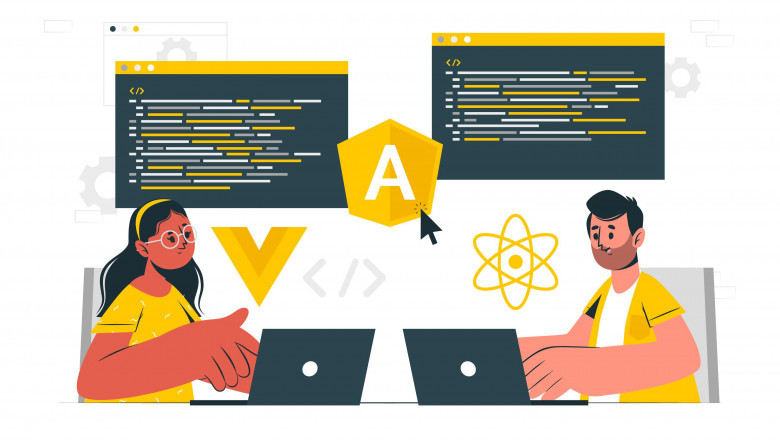
Have you ever wanted to build a mobile app? Are you frustrated with the seemingly endless steps of coding, compiling, and testing that goes into every app project? If so, this blog article is for you!
Recent Statistics on React Native App Development
React Native is a JavaScript framework that allows you to create native mobile apps. It's based on React, Facebook's JavaScript library for creating user interfaces, but instead of being aimed at browsers, it's aimed at mobile platforms.
In the past year, React Native has become one of the most popular frameworks for building native mobile apps. This is due in part to its ease of use and ability to create cross-platform apps.
According to a recent report from App Annie, React Native was the third most popular platform for developing new iOS applications in Q1 2019. It was also the fifth most popular platform for developing new Android applications in Q1 2019.
The popularity of React Native is also evident in GitHub's annual Octoverse report. In 2018, React Native was the 15th most popular repository on GitHub with over 24,000 stars. That placed it ahead of other popular repositories such as Vue.js and AngularJS.
With its popularity on the rise, there are sure to be more statistics released in the coming months about React Native app development.
What Is React Native?
React Native is a cross-platform mobile app development framework that lets you create native apps for Android and iOS using React. If you're a web developer looking to branch out into mobile app development, React Native is an excellent choice. It lets you use your existing knowledge of React to build native apps without having to learn a new language or framework.
React Native also has the advantage of being open source, so there's a large community of developers who are always working on improving it.
If you're interested in learning more about React Native, check out our complete guide to creating a React Native app.
React Native Components
There are two types of components in React Native:
Functional components
Functional components are simple and easy to write. They are basically JavaScript functions that accept props and return JSX. We can use them for small, one-off pieces of UI, like buttons or simple presentational components.
Class-based components
Class-based components are more complex and allow us to use features like local state and lifecycle methods. They are usually used for larger pieces of UI, like forms or sections of our app.
Building a React Native App
Assuming that you already have React Native installed on your system, we can now create a new project.
Step1
To do so, launch the terminal and type the following commands:
react-native init name_of_your_app
cd name_of_your_app
react-native run-ios
This will create a new React Native app with the given name and start it in the iOS simulator. You can also use 'react-native run-android' to start the app in the Android emulator.
Step 2
Now that the project is set up, we can start building our app.
The first thing we need to do is import the React Native components that we will be using. For this example, we will be using the 'View' and 'Text' components.
import React, { Component } from 'react';
import { View, Text } from 'react-native';
Next, we need to create a class that extends the 'Component' class. This class will be responsible for rendering our app's UI.
class App extends Component {
render() {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text>Hello World!</Text>
</View>
);
}
}
export default App;
We need to export our App class so that it can be used by other parts of our app.
Step 3
Now that we have our App class setup, we can use it to render our app's UI. To do this, we need to open the 'App.js' file and add the following code:
import React, { Component } from 'react';
import { View, Text } from 'react-native';
class App extends Component {
render() {
return (
<View>
<Text>Hello World!</Text>
</View>
);
}
}
export default App;
This code will render the 'Hello World!' text to the screen. If you run the app now, you should see the following output:
Hello World!
Testing and Deploying Your App
Assuming you have followed all of the previous steps in this guide, you are now ready to test and deploy your app.
To test your app, you will need to run it on a physical device or an emulator. The process for doing this will vary depending on your platform (iOS or Android).
Once you have successfully tested your app on a physical device or an emulator, you are ready to deploy it. Deploying an app is different from testing an app because it makes the app available to the general public.
There are many ways to deploy a React Native app, but the most common method is to use Expo. Expo is a platform that allows you to create and publish React Native apps with ease.
Resources
If you're looking to create a React Native app, you're in luck! There are numerous excellent resources available to assist you in getting started.
We'll go over some of the best resources for learning how to create a React Native app. We'll cover books, online courses, and more. By the end, you'll be ready to start building your own app!
Books
If you like to learn from books, there are several great options available for learning React Native. Here are some of the most preferred:
- React Native in Action by Nader Dabit
- Learning React Native by Bonnie Eisenman
- Building React Native Apps by Jonathan Fielding
Online Courses
If you prefer learning through online courses, there are some great options available as well. Here are some of the most preferred:
- The Complete React Native + Hooks Course by Stephen Grider
- React Native: Advanced Concepts by Colin Eberhardt -Get Started with Reactive programming and learn RxJS observables from scratch! By Andrei Neagoie
Whichever resource you choose, you'll be on your way to becoming a React Native expert in no time!
Summing Up
React Native is an amazing framework for creating mobile applications. It's easy to use and helps you create high-quality apps quickly. In this guide, we've shown you how to create a React Native app from start to finish with a basic example. We hope you found it helpful!