views
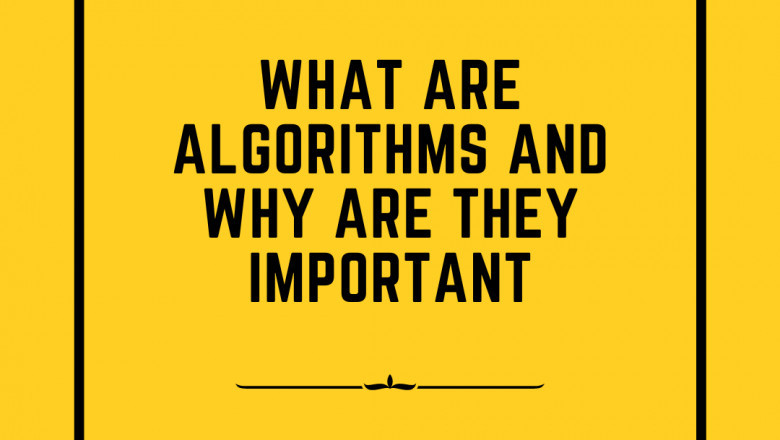
What are Algorithms and Why Are They Important
The word "algorithm" is commonly used in coding and computer science. What is it, and why is it necessary for coding? In this article, I’ll explain what an algorithm is and why they are important in every aspect of the programming field.
What is an algorithm?
An algorithm is a set of instructions/ rules to adhere to, for carrying out a particular task or resolving a particular issue. There are algorithms everywhere around us. An algorithm is used, for instance, in the process of doing laundry, baking a cake, and solving a long division issue. What might a list of instructions for baking a cake look like, similar to an algorithm?
-
Warm the oven up.
-
assemble the components
-
Count the components.
-
To prepare the batter, combine the ingredients.
-
Oil a pan.
-
Fill the pan with the batter.
The main goal of algorithmic programming is to create a set of instructions that tell the computer how to carry out a task. A computer program is essentially an algorithm that instructs the machine how to carry out a certain task by telling it what exact actions to take and in what order.
Types of Algorithms
The ideas that algorithms employ to complete a task are used to categorize them. Although there are many different kinds of algorithms, the following are the most basic kinds:
-
Algorithms that "divide and conquer" the problem by breaking it down into smaller subproblems of the same type, solving those, and then combining those solutions to solve the original problem.
-
Try every potential solution using brute force approaches until one is identified.
-
Randomized algorithms - Find a solution to the problem by using a random number at least once throughout the computation.
-
Greedy algorithms - Discover the best possible answer locally, intending to find the best possible answer globally. For detailed information, you can check out the popular data structure and algorithms course, by Learnbay.
Example of an Algorithm
-
Figuring out a Rubik's cube
There are numerous strategies for solving a Rubik's cube, ranging in complexity from extremely straightforward to quite complex. Here is simply one straightforward algorithm. Let's first choose a notation (similar to a programming language).
The first letter of each of a Rubik's cube's six faces can be used to represent that face:
-
U - up
-
D - down
-
L - left
-
R - right
-
F - front
-
B - back
There are three possible rotations for each face. Using U as an illustration, these are shown:
-
U - quarter-turn of the upper face in a clockwise direction
-
U' - quarter-turn of the upper face in the opposite direction.
-
U2 - half turn of the upper face in either direction
Let's now walk through the algorithmic processes required to solve a Rubik's Cube. Take one of your own and follow along if you like!
Step 1: The Cross
-
First, flip certain edges such that the upper face has a white cross.
-
Turns F, R', D', R, F2, R', U, R, U', R', R2, L2, U2, R2, L2 should be applied.
-
Now the cross can be solved.
Step 2: The White Corners
-
The corners are still there, but the edges of the white face are finished.
-
Apply one of the following series of turns, depending on which corner of the problem is white, orange, or green:
-
R, D, R, D at the bottom (repeat until the corner moves to its correct place)
-
R', D', R, and D (this moves the corner to the bottom; then follow the above instructions)
Step 3: Middle Layer Edges
-
The white side should be on the bottom as you flip the cube.
-
Keep an eye out for an edge on the top face that isn't yellow.
-
Make a U-turn such that the front face of the edge and the center have the same hue.
Step 4: Yellow Cross
-
Apply the following turns until a cross with a yellow center appears on the face: F, R, U, R', U, F'.
-
Apply the turns F, U, R, U', R, F' if there is an "L" shape and the two yellow pieces showing are next to one another.
-
Apply the following turns if there is a horizontal "Line" shape: F, R, U, R', U, F'.
Step 5: Sune and Antisune
-
Look at the face that has a yellow center.
-
Apply one of the series of turns below depending on the circumstances below:
-
When there is just one orientated corner: R', U', R", U', R', U2 (repeat until the desired position is attained)
-
There is one corner facing left and one facing right: U2, R, U2, R', U', R, U', R'.
Step 6: Finishing the puzzle
-
Look for "headlights" in groups (two stickers of the same color in the same row, separated by a sticker of a different color).
-
Apply one of the sequence of turns below, based on how many there are:
-
If each side has a set of headlights: R', U', R', U', R2, R, U', R, U,
-
In the absence of that, R', F, R', B2, R, F', R', B2, R2.
Sorting Algorithms
-
An algorithm for sorting arranges the items in a list in a certain order, typically in numerical or lexical order. Sorting is frequently a crucial initial step in algorithms that address more challenging issues. There are many different sorting algorithms, and each has advantages and disadvantages.
Where are Algorithms Used in Computer Science?
Each and every aspect of computer science uses algorithms. They are the foundation of the field. In computer science, an algorithm provides the computer with a precise set of instructions that enables the computer to perform any task, such as controlling a rocket or a calculator. At their heart, computer programmes are simply algorithms encoded in computer-readable programming languages.
Why are Algorithms Important to Understand?
In many different professions, the ability to specify precise methods to address a problem—a skill known as algorithmic thinking—is essential. Students that use algorithmic thinking can dissect issues and visualize fixes as distinct processes.
Are you looking for resources to master Algorithms, Sign up for the best DSA training in India and become an expert in Data structures and algorithms.