views
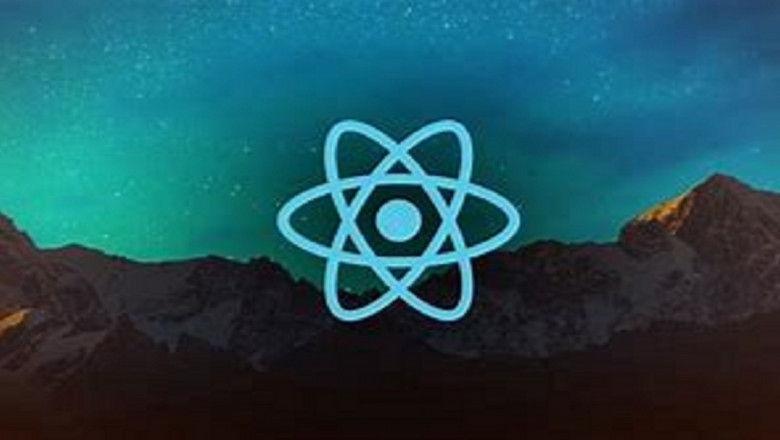
Introduction
Hooks are here to aid you if you don't like lessons. Hooks are methods that let you leverage React's state and lifecycle capabilities without having to use classes. It allows you to connect into React state and lifecycle features from function components, as the name implies.
Hooks don't work inside classes and are backwards compatible, which means they don't introduce any new features. So it's entirely up to you whether you want to use them or not. This new functionality allows you to leverage all of React's features, including function components.
Make sure your hooks are written in standard follow format. To ensure that hooks are called in the same sequence every time, call them at the top level of React functions. Avoid calling them from inside loops, nested functions, or conditions. Also, make sure you're calling them from a React function component rather than a JavaScript function. If you don't follow that guideline, you can end up with some unusual behaviours. To ensure that these rules are applied automatically, React includes a linter plugin.
To use hooks, you don't need to install anything. They're included with React starting with version 16.8.
To set up the React development environment (you'll need the newest version of Node.js to run npx), follow these steps:
npx create-react-app exploring-hooks
React hooks have rendered render props and HOCs (Higher-Order Components) practically unnecessary, making sharing stateful code much more pleasant.
React comes with a number of hooks pre-installed. The most important are useState and useEffect.
Need for React Hooks
-
To reuse stateful logices: The render props pattern and higher-order components (HOC) are two advanced React ideas. It is not possible to reuse stateful component logic in React in any way. Although the use of HOC and render properties patterns can solve this problem, it makes the code base inefficient and harder to understand because components are wrapped in a slew of others to share functionality. Hooks allow us to exchange stateful functionality in a much better and cleaner way without altering the component hierarchy.
-
‘This’ keyword :The keyword 'this' is used for two reasons. The first has nothing to do with React and everything to do with javascript. To work with classes, we must first understand how the javascript 'this' keyword works, which is rather different from other languages. Although the principles of props, state, and unidirectional data flow are simple to grasp, using the 'this' keyword when implementing class components might be challenging. Event handlers must also be bound to the class components. Classes have been discovered to not concise efficiently, leading in unreliable hot reloading, which can be remedied with Hooks.
-
Simplifying challenging scenarios: When designing components for complicated scenarios like data fetching and event subscription, all relevant code is likely to be scattered throughout multiple life cycle methods.
Effect Hook
We use the Effect hook, i.e. useEffect, from React function components to conduct side effects tasks like modifying the DOM, data fetching, and so on. These actions are so named because they can have an impact on other components and aren't possible to perform during rendering. componentDidMount, componentDidUpdate, and componentWillUnmount are all powered by the UseEffect hook. Because the components require access to their state and props, UseEffect is declared inside them. UseEffect can be called at the beginning of a render, at the end of each render, or to specify clean up depending on how we express them. They run by default after every render, including the first one.
import React, {
useState,
useEffect
} from 'react';
function usingEffect() {
const [count, setCount] = useState(0);
/* default behaviour is similar to componentDidMount and componentDidUpdate: */
useEffect(() => {
// Change document title
document.title = `Clicked {count} times`;
});
return (
<div>
<p>Clicked count = {count}</p>
<button onClick={() => setCount(count + 1)}>
Button
</button>
</div>
);
}
The document title is updated every time the count is updated in the example above. Because it runs at the first render and after every update, useEffect works similarly to componentDidMount and componentDidUpdate combined. This is useEffect's default behaviour, although it can be altered. Let's have a look at how.
If you want to use useEffect as componentDidMount then you can pass an empty array [] in the second argument to useEffect as in the below example:
import React, {
useState,
useEffect
} from 'react';
function usingEffect() {
const [count, setCount] = useState(0);
// Similar to componentDidMount
useEffect(() => {
// Only update when component mount
document.title = `You clicked {count} times`;
}, []);
return (
<div>
<p>Your click count is {count}</p>
<button onClick={() => setCount(count + 1)}>
Button
</button>
</div>
);
}
The useState() function component is used to define, alter, and use states using hooks. The current state and a function to update the state are returned as two value pairs. It's something along these lines. This is not the same as setState in a class. The fact that setState does not merge the old and new states distinguishes it. UseState() is a function component that can be called from within a function component or from an event handler. The initial state is supplied as an input to useState(), and it is used on the first render. There are no restrictions on the number of state hooks that can be used in a single component; we can use as many as we wish.
import React, {
useState
} from 'react';
function usingStateHook() {
// use useState() to declare state variable count
const [count, setCount] = useState(0);
return (
<div>
{/* display count value */}
<p>Your click count is {count}</p>
{/* on click update count value using setCount */}
<button onClick={() => setCount(count + 1)}>
Button
</button>
</div>
);
}
In the above example, we've included a counter that keeps track of how many times the button has been pressed. In this case, useState(0) sets the count to '0' and returns the setCount function, which can be used to update the count. In the onClick event handler, we call setCount with the value 'count+1'. Here, count is the previous count value; for example, if the count was two before, setCount will increase it by one to three.
Custom Hooks
React also allows us to create our own hook, which is a javaScript function with the word 'use' at the beginning of its name and can call other hooks. When we have parts that can be reused in our project, we normally develop custom hooks. We must first define them, which may include the use of other hooks, before we can refer to them as other hooks.
function FriendStatus(props) {
const isOnline = useFriendStatus(props.friend.id);
if (isOnline === null) {
return 'Loading...';
}
return isOnline ? 'Online' : 'Offline';
}
function FriendListItem(props) {
const isOnline = useFriendStatus(props.friend.id);
return (
<li style={{ color: