views
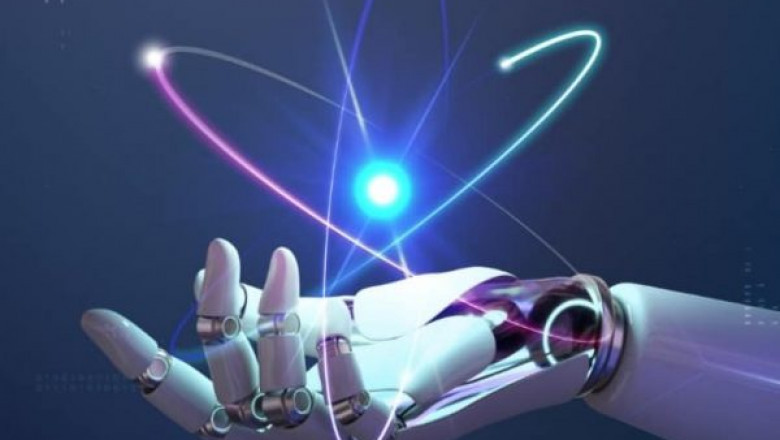
Introduction To Python Data Structures
Data structures are a means to arrange data such that, depending on the situation, it can be retrieved instantly. The data structure is any programming language's essential building block upon which a programme is based. Compared to other programming languages, Python makes it easier to master the fundamentals of these data structures.
This article will discuss the Python programming language's data structures and how they relate to some particular Python data types. We'll talk about all the built-in data structures, such as list tuples and dictionaries, as well as some more complex ones, like trees and graphs.
-
Lists
Python lists are exactly like arrays, which are stated in other languages and are collections of data in an ordered fashion. Since a list's items don't have to be of the same type, it is immensely flexible.
Python's version of lists is comparable to C++'s vectors or Java's array lists. As all the components must be relocated, adding or removing a member from the list's beginning is the most expensive operation. If the preallocated memory is all used up, insertion and deletion at the end of the list may also become expensive.
-
Dictionary
A Python dictionary has the same time complexity as an O hash table in any other language (1). Dictionary is a data type that stores a key: value pair as opposed to other data types, which only carry a single value as an element. It is used to store data values similar to a map. Key-value pairs are included in the dictionary to increase its efficiency.
With the use of keys, the Python Dictionary is indexed. They can be of any hashable type, i.e., a constant object like a text, number, tuple, etc. Using curly brackets () or dictionary comprehension, we can build a dictionary. Get a detailed explanation of Python DSA, through the best DSA training by Learnbay.
-
Tuple
A Python tuple is a collection of Python objects, similar to a list, except tuples are immutable by nature. Once they are generated, their elements cannot be changed or added to. Like a List, a Tuple can have elements of many types.
Tuples are created in Python by combining a series of values separated by a "comma," either with or without using parenthesis.
-
Set
An unordered, dynamic data collection called a Python Set bans duplicate elements. Sets are mainly employed for membership screening and the elimination of duplicate entries. This uses the widely used Hashing data structure, which typically traverses, inserts, and deletes data in O(1) time.
A Linked List is generated by attaching the value to the index point when several values are at the same location. The implementation of CPython Sets uses a dictionary of dummy variables, with the key being the set members with the highest time complexity optimizations.
-
Frozen Sets
Python has methods and operators that give results without changing the frozen set or sets to which they are applied and are immutable objects known as frozen sets. While a set's components can be changed at any moment, a frozen set's components don't change after it has been created.
-
String
Unicode characters are represented as arrays of bytes in Python Strings. A string can be thought of as an immutable collection of characters. A single character in Python is just a string of length 1 since there is no such thing as a character data type.
-
Bytearray
A modifiable sequence of integers in the range of 0 to x to 256 is provided by the Python bytearray.
We have so far covered every data structure that is a part of the primary Python language. They are essential to learn if you’re a developer. Head over the popular data structures and system design course, and master the Python DSA from scratch.